图像处理与分析——实验六-数学形态及图像压缩
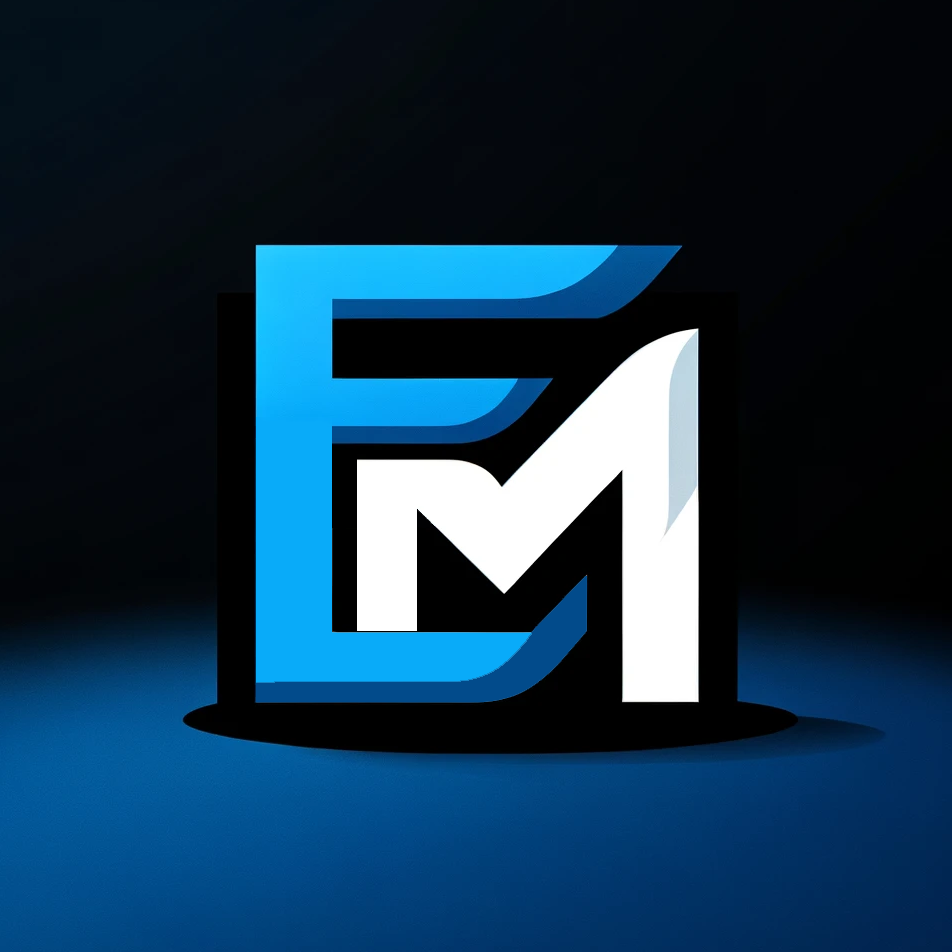
实验目标:
- 掌握图像压缩和数学形态学基本方法及技术。
- 使用不同参数进行图像压缩测试,观察其效果。
- 通过形态学操作提取图片中的特定图案。
一、
实验要求:
- 对 imgs 目录下的图像进行图像压缩测试,调节参数查看效果。
实验图片路径为:
imgs/1.jpg
imgs/2.jpg
imgs/3.jpg
输出路径为:outputs/
请按照 exp5_1_i 的格式,输出每个任务结果
实验方案:
图像读取与预处理:
- 使用
mpimg.imread
从指定路径读取图像,并转换为 numpy 数组以便进行进一步处理。
1
2
3
4
5
6
7
8
9
10import os
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
def load_image(path):
return mpimg.imread(path)
def save_image(image, path):
plt.imsave(path, image)- 使用
图像压缩:
- 通过改变图像分辨率来“压缩”图像。
- 使用一个缩放因子,将图像的高度和宽度按比例缩小。
1
2
3
4
5
6
7
8def compress_image(image, scale_factor=0.5):
height, width = image.shape[:2]
new_height, new_width = int(height * scale_factor), int(width * scale_factor)
compressed_image = np.zeros((new_height, new_width, image.shape[2]), dtype=image.dtype)
for i in range(new_height):
for j in range(new_width):
compressed_image[i, j] = image[int(i / scale_factor), int(j / scale_factor)]
return compressed_image图像显示与保存:
- 显示原图和压缩后的图像对比,并将结果保存到指定路径。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26def display_images(original, compressed, output_path):
fig, axes = plt.subplots(1, 2, figsize=(10, 5))
axes[0].imshow(original)
axes[0].set_title('Before')
axes[0].axis('off')
axes[1].imshow(compressed)
axes[1].set_title('After')
axes[1].axis('off')
plt.savefig(output_path)
plt.show()
def process_images():
input_dir = "imgs"
output_dir = "outputs"
os.makedirs(output_dir, exist_ok=True)
for i in range(1, 4):
path = f"{input_dir}/{i}.jpg"
image = load_image(path)
compressed_image = compress_image(image, scale_factor=0.5)
output_path = f"{output_dir}/exp5_1_{i}.jpg"
display_images(image, compressed_image, output_path)
process_images()
实验结果:
- 原图特点:
- 图像展示了不同的场景或物体,具有较高的分辨率和清晰度。
- 处理后的图像:
- 经过压缩处理后,图像的分辨率明显降低,但大致轮廓和主要细节依然可见。
- 不同的图像在压缩后,质量有所差异,这与原图像的复杂度和压缩参数有关。
分析:
- 通过调整缩放因子,可以控制图像压缩后的质量和文件大小。
- 在实际应用中,需要根据具体需求平衡图像质量和存储空间。
二、
实验要求:
- 尝试使用形态学操作的方法提取图片 morphology.jpg 中的国旗图案。
实验图片路径为:
imgs/morphology.jpg
输出路径为:outputs/
请按照 exp5_2_i 的格式,输出每个任务结果
实验方案:
图像读取与预处理:
- 使用
cv2.imread
读取图像,并转换为灰度图。
1
2
3
4
5import cv2
import numpy as np
img = cv2.imread('imgs/morphology.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)- 使用
边缘检测与形态学操作:
- 应用高斯模糊去除噪声,并使用 Canny 边缘检测算法检测边缘。
- 使用形态学操作闭合边缘。
1
2
3
4blurred = cv2.GaussianBlur(gray, (3, 3), 0)
edges = cv2.Canny(blurred, 50, 150)
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
closed = cv2.morphologyEx(edges, cv2.MORPH_CLOSE, kernel)轮廓检测与国旗提取:
- 查找轮廓,并筛选出接近国旗比例的轮廓。
- 将提取的国旗图案进行统一大小处理,并保存结果。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22contours, _ = cv2.findContours(closed.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
flags = []
standard_flag_size = (90, 60)
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
aspect_ratio = w / float(h)
if 1.4 < aspect_ratio < 1.8:
flag = img[y:y+h, x:x+w]
resized_flag = cv2.resize(flag, standard_flag_size)
flags.append(resized_flag)
combined_height = (len(flags) // 10 + 1) * standard_flag_size[1]
combined_width = 10 * standard_flag_size[0]
combined_image = np.zeros((combined_height, combined_width, 3), dtype=np.uint8)
for idx, flag in enumerate(flags):
row = idx // 10
col = idx % 10
combined_image[row*standard_flag_size[1]:(row+1)*standard_flag_size[1], col*standard_flag_size[0]:(col+1)*standard_flag_size[0]] = flag
cv2.imwrite('outputs/exp5_2_1.jpg', combined_image)
实验结果:
- 原图特点:
- 图像包含多个国旗图案和其他复杂背景元素。
- 处理后的图像:
- 通过形态学操作,成功提取出大部分国旗图案,并将其拼接到一张图像中。
- 提取出的国旗图案清晰可见,背景杂乱的部分被有效去除。
分析:
- 形态学操作在提取特定形状和结构元素方面非常有效,特别是当目标对象具有明显的几何特征时。
- 大约有30%的国旗未能被提取出来,可能是由于这些国旗在图像中的位置、大小或形状与预设参数不完全匹配。
实验总结:
本次实验主要探索了图像压缩和数学形态学操作两种技术。在第一项任务中,通过调整压缩参数,实现了不同质量的图像压缩效果。这一过程展示了图像压缩技术在实际应用中的灵活性和重要性。
在第二项任务中,使用形态学操作成功提取出图像中的国旗图案。这一过程不仅展示了形态学操作的强大功能,还加深了对图像结构分析和处理技术的理解。
通过本次实验,我不仅掌握了图像压缩和形态学操作的基本方法,还学会了如何在实际应用中灵活运用这些技术,以解决不同类型的图像处理问题。这些经验和技能将为未来更加复杂的图像处理任务提供有力支持。
- Title: 图像处理与分析——实验六-数学形态及图像压缩
- Author: ELecmark
- Created at : 2024-05-30 23:50:13
- Updated at : 2024-06-01 00:12:17
- Link: https://elecmark.github.io/2024/05/30/图像处理与分析——实验六-数学形态及图像压缩/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments